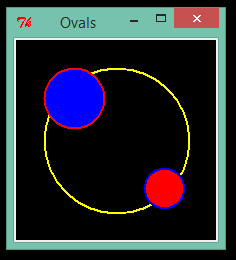
The first code is a simple example:
import Tkinter root = Tkinter.Tk() ch, cw = 200, 200 c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black") c1.create_oval(30, 30, 175, 175, outline = "yellow", width = 2) c1.create_oval(30, 30, 90, 90, outline = "red", fill = "blue", width = 2) c1.create_oval(130, 130, 170, 170, outline = "blue", fill = "red", width = 2) c1.pack() root.title("Ovals") root.mainloop()
We must create a canvas using the "Canvas" method, also its dimensions are declared here.
c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black")
Then we create an oval by using:
c1.create_oval(30, 30, 175, 175, outline = "yellow", width = 2)
The coordinates of the first point are (30, 30), and of the last point are (175, 175). The attributes of outline_color and width_size go with "outline" and "width", respectively, and if there is no fill color declared, the oval will be just a colored ring.
Then we could show the results in the screen by using the "pack" or "grid" methods.

The next examples are a little more complex but show something else.
Second code sample is:
import Tkinter def f(x1, y1, x2, y2): r1, b1 = "red", "blue" c1.create_oval(x1, y1, x2, y2, outline = r1, fill = b1, width = 2) root = Tkinter.Tk() ch, cw = 200, 200 c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black") for i in range(7): a = 0.5 f(30 + 3*a*i, 30 + a*i*i*(-1**i), 90 + 3*a*i, 90 + a*i*i) f(100 + 3*i, 100 + 3*i, 120 + 3*i, 120 + 3*i) f(120 + 3*i, 90 + 3*i, 125 + 3*i, 140 + 3*i) c1.pack() root.title("Ovals_abstract") root.mainloop()
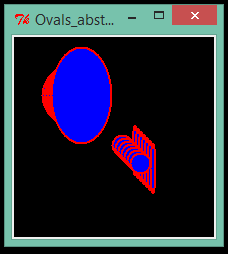
Third code sample is:
import Tkinter # line: function that draws a red line def line(a, b): x0, y0, x1, y1 = a[0], a[1], b[0], b[1] c1.create_line(x0, y0, x1, y1, fill = "red", width = 1) # n : function that draws a blue circle def n(x1, y1, x2, y2, w): r1 = "blue" c1.create_oval(x1, y1, x2, y2, outline = r1, width = w) root = Tkinter.Tk() ch, cw = 300, 300 rm = 10 A = (0.5 * cw, rm ) B = (0.5 * cw, ch - rm ) C = (rm , 0.5 * ch) D = (cw - rm , 0.5 * ch) c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black") for i in range(1, 6): if i < 4: a, w = 7, 1 else: a, w = 25, 6 n(ch * 0.5 - a*i, cw * 0.5 - a*i, ch * 0.5 + a*i, cw * 0.5 + a*i, w) a, b = ch * 0.5 - a*i, cw * 0.5 - a*i c, d = ch * 0.5 + a*i, cw * 0.5 + a*i # print i, a, b # print i, c, d line(A, B) line(C, D) n(50, 50, 250, 250, 6) c1.pack() root.title("Ovals PTR") root.mainloop()
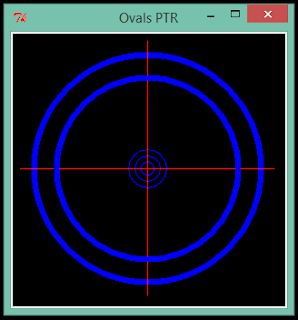
No comments:
Post a Comment