
The first code is a simple example:
from Tkinter import * root = Tk() c1 = Canvas(root, height = 180, width = 200, background = "black" ) c1.create_rectangle( 27, 17, 103, 93, fill = "blue", outline = "cyan") c1.create_rectangle(103, 93, 179, 169, fill = "red", outline = "yellow") c1.pack() root.title("Rectangles") root.mainloop()
We must create a canvas using the "Canvas" method, also its dimensions are declared here.
c1 = Canvas(root, height = 180, width = 200, background = "black" )
Then we create a rectangle using:
c1.create_rectangle( 27, 17, 103, 93, fill = "blue", outline = "cyan")
The coordinates of the first point are (27, 17), and of the last point are (103, 93). The attributes of fill_color and outline_color go with "fill" and "outline", respectively.
Then we could show the results in the screen by using the "pack" or "grid" methods.
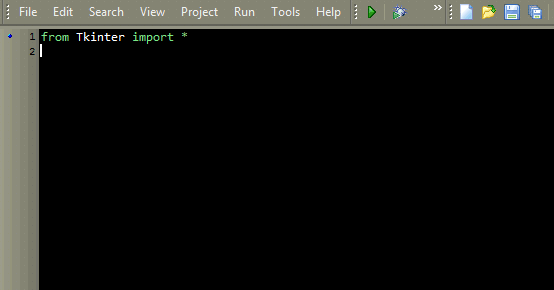
The next example has many lines of code but show something else than just a couple of rectangles.
Second code sample is:
import Tkinter def line(a, b, color, w): x0, y0, x1, y1 = a[0], a[1], b[0], b[1] c1.create_line(x0, y0, x1, y1, fill = color, width = w) def rect(a0, b0, outlin3, fill_, width_): a, b, c, d = a0[0], a0[1], b0[0], b0[1] o0, f0, w0 = outlin3, fill_, width_ # outline="#f11" fill="#1f1", width=2 c1.create_rectangle(a, b, c, d, outline = o0, fill = f0, width = w0) def desktop_(): A, B = [16, 76], [179, 81] rct_1 = rect(A, B, "black", "orange", 1) def screen_(): A, B = [ 68, 20], [156, 64] C, D = [ 70, 23], [153, 61] E, F = [ 89, 73], [135, 76] G, H = [110, 64], [114, 73] rct_1 = rect(A, B, "black", "green", 1) rct_1 = rect(C, D, "black", "black", 1) rct_1 = rect(E, F, "black", "green", 1) rct_1 = rect(G, H, "black", "green", 1) def speakers_(): A, B = [ 52, 60], [ 64, 76] C, D = [160, 60], [172, 76] E, F = [ 54, 62], [ 62, 74] G, H = [162, 62], [170, 74] rct_1 = rect(A, B, "black", "green", 1) rct_1 = rect(C, D, "black", "green", 1) rct_1 = rect(E, F, "black", "gray", 1) rct_1 = rect(G, H, "black", "gray", 1) def cpu_(): A, B = [ 24, 28], [ 47, 76] C, D = [ 33, 47], [ 37, 51] E, F = [ 40, 48], [ 42, 50] G, H = [ 28, 48], [ 30, 50] I, J = [ 27, 39], [ 30, 42] K, L = [ 34, 39], [ 37, 42] M, N = [ 40, 39], [ 44, 42] O, P = [ 27, 31], [ 44, 36] Q, R = [ 27, 57], [ 44, 72] rct_1 = rect(A, B, "black", "green", 1) rct_1 = rect(C, D, "black", "black", 1) rct_1 = rect(E, F, "blue", "blue", 1) rct_1 = rect(G, H, "red", "red", 1) rct_1 = rect(I, J, "black", "black", 1) rct_1 = rect(K, L, "black", "black", 1) rct_1 = rect(M, N, "black", "black", 1) rct_1 = rect(O, P, "black", "blue", 1) rct_1 = rect(Q, R, "black", "blue", 1) root = Tkinter.Tk() ch, cw = 100, 200 c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "white") desktop_() screen_() speakers_() cpu_() c1.pack() root.title("Good Old Desktop PC") root.mainloop()

The third code sample is:
import Tkinter def line(a, b, color, w): x0, y0, x1, y1 = a[0], a[1], b[0], b[1] c1.create_line(x0, y0, x1, y1, fill = color, width = w) def pattern_1(): sx = sy = -20 dx = dy = 50 """ First Wave """ for j in range(3): for i in range(3): a = [sx + (1 + 2*i) * dx , sy + (1 + 2*j) * dx ] b = [a[0] + dx, a[1] + dx] rect(a, b, "white", "mediumseagreen", 3) """ Second Wave """ a2 = [sx + 1.5 * dx , sy + 1.5 * dx] b2 = [a2[0] + 4 * dx, a2[1] + 4 * dx] rect(a2, b2, "white", "mediumseagreen", 3) root = Tkinter.Tk() ch, cw = 300, 300 c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black") pattern_1() c1.pack() root.title("SQ Pattern") root.mainloop()
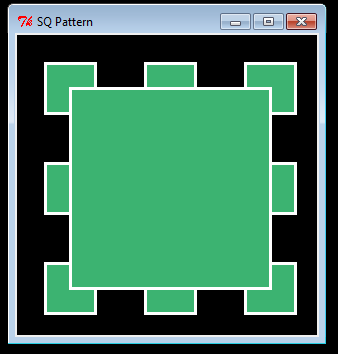
No comments:
Post a Comment