
The first code is a simple example:
import Tkinter root = Tkinter.Tk() ch, cw = 170, 170 c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black") ps = [ 60, 130, 40, 120, 40, 80, 70, 40, 100, 40, 130, 80, 130, 120, 110, 130] c1.create_polygon(ps, outline = 'yellow', fill = 'teal', width = 2) c1.pack() root.title("BckP") root.mainloop()
We must create a canvas using the "Canvas" method, also its dimensions are declared here.
c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = "black")
Then we create a polygon by using:
c1.create_polygon(points, outline='yellow', fill='teal', width=2)
The coordinates of the points that define the polygon are in the "ps" list:
ps = [ 60, 130, 40, 120, 40, 80, 70, 40,
100, 40, 130, 80, 130, 120, 110, 130]
Then we could show the results in the screen by using the "pack" or "grid" methods.
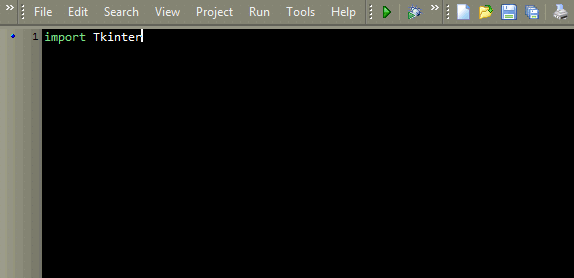
The next examples have some variations.
Second code sample is:
import Tkinter root = Tkinter.Tk() ch, cw = 200, 200 a, b = 40, 40 bgc = "blue" # bgc : background colour cr1 = "brown" cr2 = "yellow" c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = bgc) points = [ 60 + a, 130 + b, 40 + a, 120 + b, 40 + a, 80 + b, 70 + a, 40 + b, 100 + a, 40 + b, 130 + a, 70 + b, 2 + a, 0 + b, 0 + a, 0 + b] c1.create_polygon(points, outline = cr1, fill = cr2, width = 2) c1.pack() root.title("Dmd01") root.mainloop()
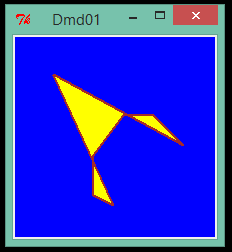
Third code sample is:
import Tkinter def tr(a, b, m, n): ax, ay = [ 0 * m + a, 0 * n + b] bx, by = [ 0 * m + a, 20 * n + b] cx, cy = [17 * m + a, 10 * n + b] ps = [ax, ay, bx, by, cx, cy] c1.create_polygon(ps, outline = cr1, fill = cr2, width = 2) root = Tkinter.Tk() s = 20 ch, cw = 200, 200 a, b, n = 40, 30, 14 bgc = "black" # bgc : background colour cr1 = "white" cr2 = "red" c1 = Tkinter.Canvas(root, height = ch, width = cw, bg = bgc) # Lil' triangles array for i in range(17): tr(20 + 10 * i, 20, 0.5, 0.5) tr(20 + 10 * i, 180, 0.5, 0.5) # Parts of a big triangle tr(45 + s, 45, 2.5, 2.5) tr(95 + s, 75, 2.5, 2.5) tr(45 + s, 105, 2.5, 2.5) c1.pack() root.title("TrG1") root.mainloop()
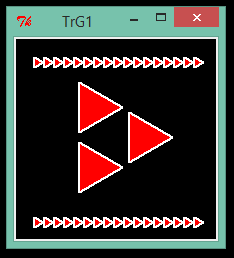
No comments:
Post a Comment