
Code for the simple Mach Number calculator:
from Tkinter import * def mc(): # 1st Function try: ns00, ns01 = a00.get(), a01.get() ns02, ns03 = a02.get(), a03.get() V = ns00 k = ns01 R = ns02 T = ns03 """ M : Mach Number """ M = V / (k * R * T) ** 0.5 a = "M = " + "{:10.10f}".format(M) except ZeroDivisionError: a = "Zero Div Error" print "Zero Division Error" except ValueError: a = "Value Error" print "Value Error" ns1.config(text = a) ns1.grid(row = 5, column = 1, sticky = W) def ent(var, row, col, width = None): # 2nd Function w = Entry(root, textvariable = var, width = 19) w.grid(row = row, column = col) return w def tx7(x, y, txt): # 3rd Function w = c1.create_text(x, y, anchor = W, font="Impact", text = txt) return w def txt(x, y, txt, size, colour): c1.create_text( x, y, anchor = W, font = ("Verdana", size), text = txt, fill = colour) def line(x0, y0, color, x1, y1): # 4th Function w = c1.create_line(x0, y0, x1, y1, fill = color) return w def btn(txt, row, col, cmd = None): # 5th Function w = Button(root, text = txt, command = cmd) w.grid(row = row, column = col) return w def lab(txt, row, col): # 6th Function w = Label(root, text = txt) w.grid(row = row, column = col) return w def frame_c(c1, c2): # 7th Function line(x0, y0, c1, x3, y3), line(x0, y0, c2, x1, y1) line(x2, y2, c1, x1, y1), line(x2, y2, c2, x3, y3) root = Tk() ch, cw = 70, 200 x0, y0 = 02 , 02 x1, y1 = 02 , ch - 2 x2, y2 = cw - 2, ch - 2 x3, y3 = cw - 2, 02 c1 = Canvas(root, height = ch, width = cw) frame_c("blue", "green") a0, b0 = 5, 12 line(112 + a0, 32 + b0, "red", 116 + a0, 45 + b0) line(116 + a0, 24 + b0, "red", 116 + a0, 45 + b0) line(116 + a0, 24 + b0, "red", 165 + a0, 24 + b0) tx7(20, 30, "M"), tx7(40, 30, "=") tx7(59, 22, "_"), tx7(80, 30, "=") tx7(60, 20, "V"), tx7(60, 40, "c") tx7(144, 22, "_"), tx7(125, 49, "k") tx7(140, 20, "V"), tx7(140, 49, "R") tx7(157, 49, "T"), tx7(137, 22, "_") tx7(130, 22, "_"), tx7(121, 22, "_") tx7(151, 22, "_"), tx7(158, 22, "_") tx7(114, 22, "_"), tx7(165, 22, "_") c1.grid(row = 0, column = 0, columnspan = 3) ''' 0,0 0,1 0,2 1,0 1,1 1,2 2,0 2,1 2,2 3,0 3,1 3,2 4,0 4,1 4,2 ''' a00, a01 = DoubleVar(), DoubleVar() a02, a03 = DoubleVar(), DoubleVar() v1, k1, r1, t1, e1 = "V =", "k =", "R =", "T =", "Execute" s0, s1, s2, s3, s4 = "m/s", " ", "J/(kg-K)", " K", "<>" lab(v1, 1,0) ; ent(a00, 1,1) ; lab(s0, 1,2) lab(k1, 2,0) ; ent(a01, 2,1) ; lab(s1, 2,2) lab(r1, 3,0) ; ent(a02, 3,1) ; lab(s2, 3,2) lab(t1, 4,0) ; ent(a03, 4,1) ; lab(s3, 4,2) btn(e1, 5,0, mc); Label(root) ; lab(s4, 5,2) ns1 = Label(root) root.title("Mach Number") root.mainloop()
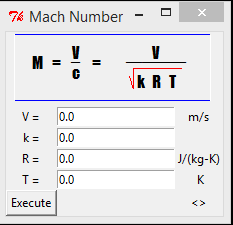
(Further reading of the Mach number is kind of easy to be found :D).
Excellent Blog! I would Thanks for sharing this wonderful content.its very useful to us.I gained many unknown information, the way you have clearly explained is really fantastic.
ReplyDeleteFull Stack Training in Chennai | Certification | Online Training Course
Full Stack Training in Bangalore | Certification | Online Training Course
Full Stack Training in Hyderabad | Certification | Online Training Course
Full Stack Developer Training in Chennai | Mean Stack Developer Training in Chennai
Full Stack Training
Full Stack Online Training
Superb article.Thanks for posting.
ReplyDeletePython Course in Nagpur
Thanks for posting this article with us . Keep Posting
ReplyDeletePython Course in Solapur
Very Informative and Helpful. Keep uploading these types of articles. Data Science Classes in Nagpur, Data Science Courses in Nagpur | Data Science Classes in Nagpur in IT Education Centre. Thank You...
ReplyDelete